Having a date with Kong, the most popular API gateway in the world
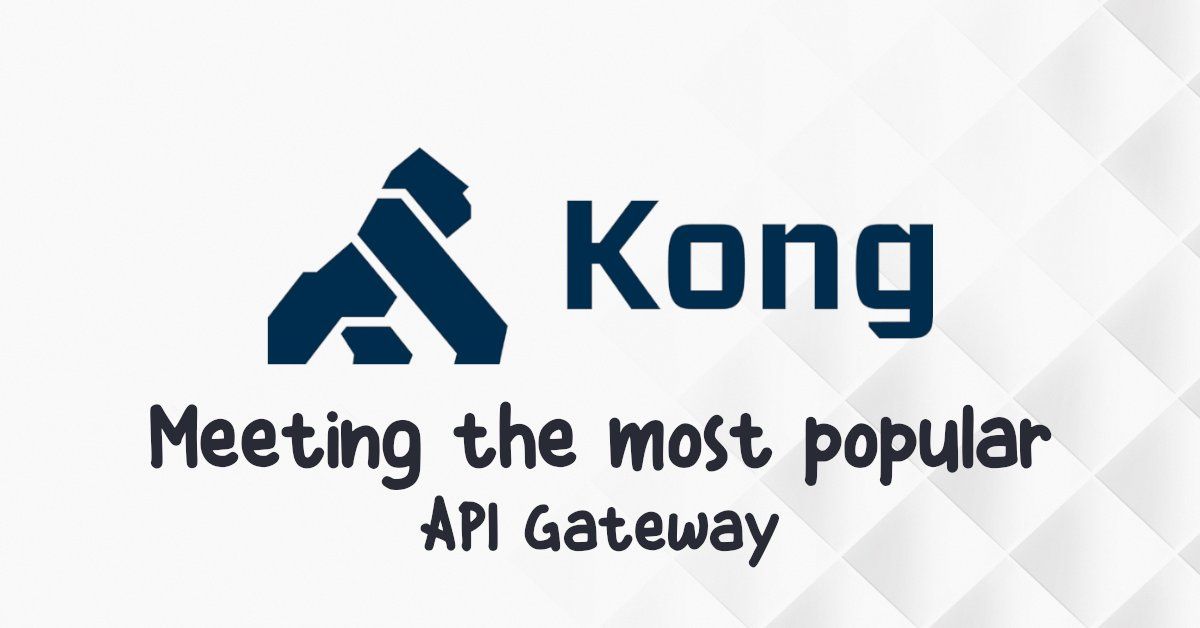
As a Developer, you must consume or provide some API in whatever context you work in. You should think about how you will handle consumers, credentials, and endpoints, and keep in mind that most frameworks cannot handle these tasks easily.
API Gateways were created to build, deploy, monitor, and orchestrate APIs. Of course, you could have tools or framework features to support this task list; evaluate what works best for your projects. To keep your focus on code and business, an API Gateway that handles Key-Auth, JWT, and OAuth2 security may be useful. To avoid complexity, tools, frameworks, and solutions should be used.
Kong has several options for database dependencies, such as Cassandra or PostgreSQL. You can use Kong without a database by using a YAML file, but some plugins that require persistence will not work. Another option is hybrid, which combines two approaches, a cluster concept with leader and worker instances.
Tell me more
Kong is built on NGINX and uses the Lua module to support plugins; however, you could now use Python, Go, and Javascript in custom plugins. Kong is used in my projects and current work.
The performance is practically identical to NGINX and can be installed in an instance with 1GB of RAM; okay, Kong takes more resources, but that's acceptable because Kong is NGINX with unlocking powers available for free.
It's important to note that Kong, like NGINX and Traefik, may be used to route Ingress Network traffic on Docker Swarm or Kubernetes.
Some useful core plugins
As I said before, you can create your custom plugins; however, if Kong does not support your need you can find community plugins. I created some public plugins based on existing plugins.
It's time to have some fun with Kong API Gateway.
The short example is a proxy from an awesome Bible API that uses key-auth to enhance security. We'll use request-transformer to bind some headers and file-log to produce some valuable access logs.
I chose the declarative approach the Kong DB-less, of course, to make things clear and avoid wasting time learning Admin API at this point.
First, we must declare our kong.yml file.
- assets/kong.yml
_format_version: "3.0"
services:
- name: my-service
url: https://labs.bible.org/api
plugins:
- name: key-auth
routes:
- name: my-route
paths:
- /
consumers:
- username: my-user
keyauth_credentials:
- key: my-key
plugins:
- name: file-log
config:
path: /tmp/api.log
- name: request-transformer
config:
add:
headers:
- content-type:application/json
- accept:application/json
Kong essentially works with services and routes, and you can define upstreams to provide health checks and load balancing when you have many entry points.
Services
Define service settings as URL or upstream and support one or more routes. Allowing plugins to be applied to all routes;
Routes
Basically, you can provide the path, query, method, and headers. Plugins can be utilized in specific cases, such as:
- Setting a rate limit for my POST creation route;
- Put specific header;
- Get logs;
Plugins
Add global plugins that will be applied for all services and routes.
Consumers
You can have one or more consumers with credentials, key-auth, OAuth2, LDAP, and other authentication methods. The consumer can be used as a plugin filter, for example:
- For the aggressive consumer, set a rate-limit of one request per hour;
- Add a particular header to track the origin of the consumer;
Now we must define your compose file.
- docker-compose.yml
version: '3.9'
services:
kong:
image: kong:3.0-alpine
environment:
KONG_LOG_LEVEL: info
KONG_ADMIN_ACCESS_LOG: /dev/stdout
KONG_ADMIN_ERROR_LOG: /dev/stderr
KONG_DATABASE: "off"
KONG_DECLARATIVE_CONFIG: /usr/local/kong/declarative/kong.yml
ports:
- "8000:8000"
- "8001:8001"
restart: unless-stopped
volumes:
- ./assets/kong.yml:/usr/local/kong/declarative/kong.yml:ro
Great job! To launch Kong API Gateway, enter the command below.
docker-compose up -d
Kong exposes two ports 8000 (proxy) and 8001 (admin API).
It's time to put Kong API Gateway to the test with the bellow command.
curl --location --request GET 'http://localhost:8000/?passage=random&type=json' \
--header 'apikey: key'
{ "message":"Invalid authentication credentials" }
Although the credentials are wrong, the security plugin works as expected.
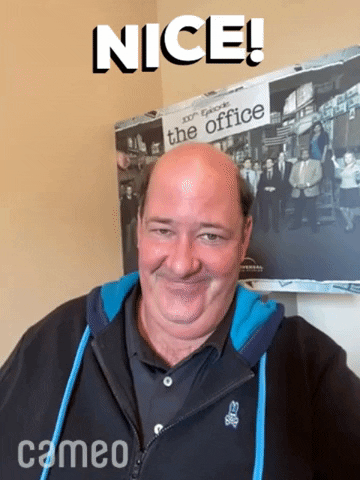
Finally, enter the correct key and wait for the API response.
curl --location --request GET 'http://localhost:8000/?passage=random&type=json' \
--header 'apikey: my-key'
[{"bookname":"Ecclesiastes","chapter":"9","verse":"1","text":"So I reflected on all this, attempting to clear it all up. I concluded that the righteous and the wise, as well as their works, are in the hand of God; whether a person will be loved or hated\u2014 no one knows what lies ahead. "}]
If you prefer, you can clone the repository.
You can also use NGINX powers to support some Kong features; please visit my related article.
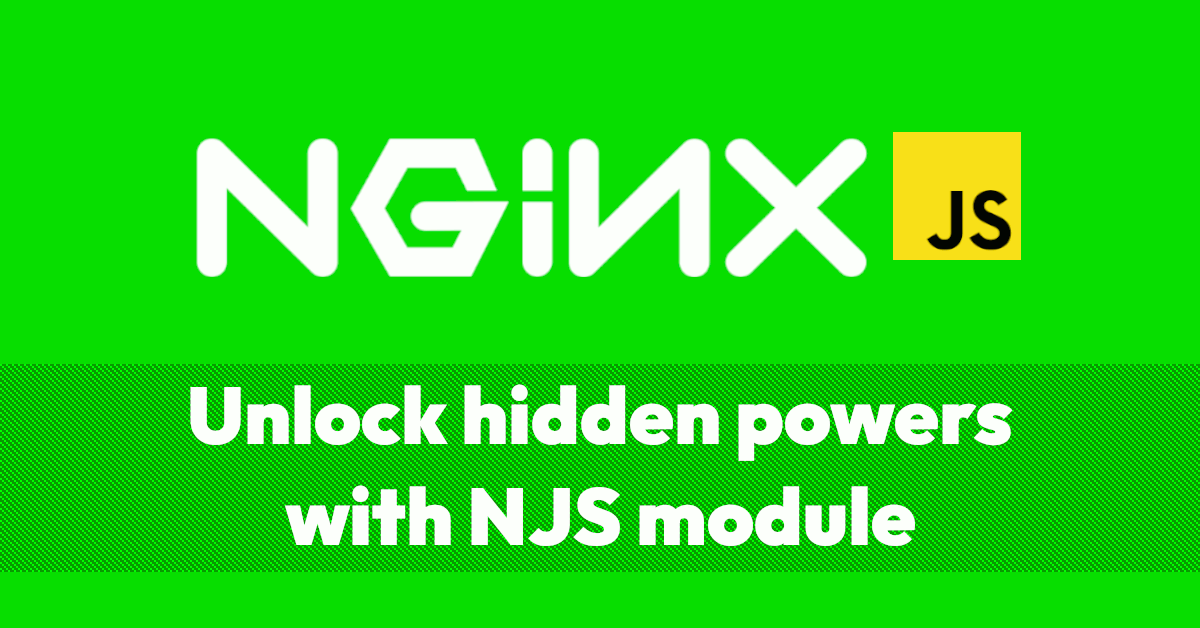
If you want to learn more about Kong's features, keep reading this article:
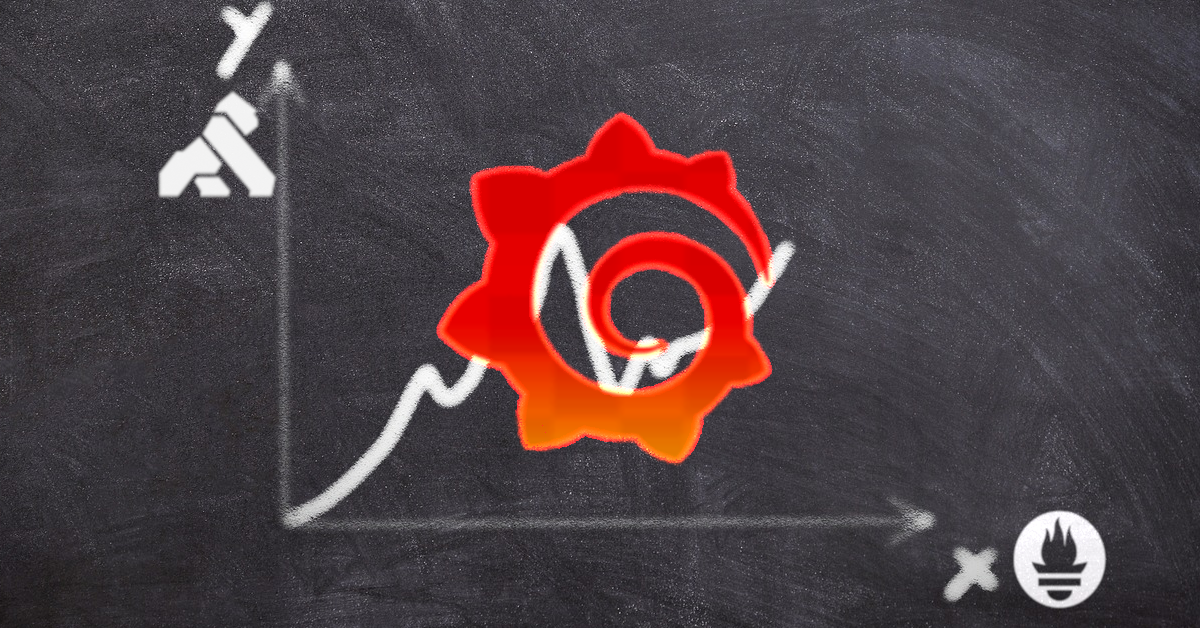
Done!
Thank you for reading; please leave comments and share your experiences to help improve the article. Now, God bless you and your kernel!
Time for feedback!