JavaScript: What is an Immediately Invoked Function Expression (IIFE)?
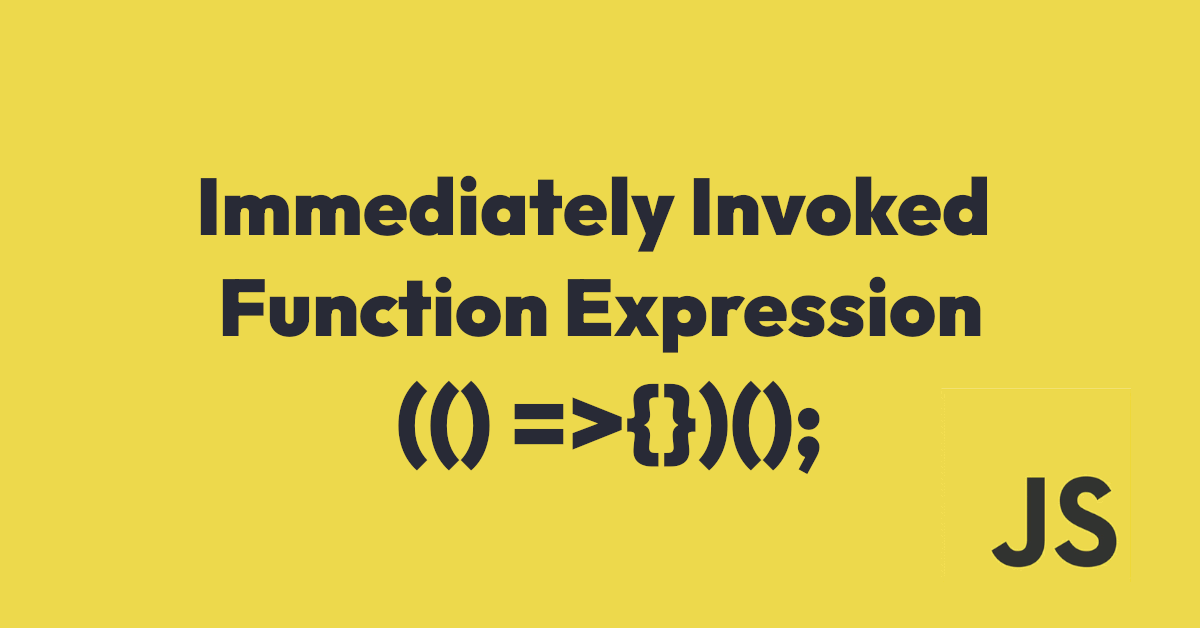
When I first saw this pattern in a book called JavaScript High Performance, I fell in love with how it was used and began to use it for everything. I had no idea about JavaScript tricks like "===", Date, "for/var", prototypes, and others at the time, but this book changed the way I wrote JavaScript code. I can say that book was worth every penny, but I now remember to land on someone and never return 😭,
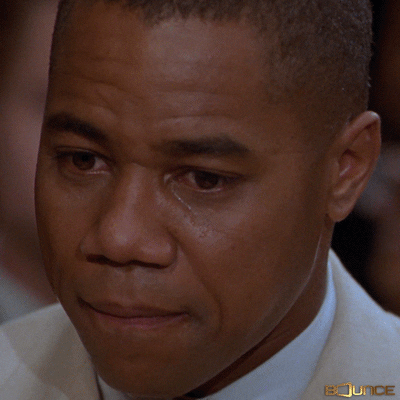
What is IIFE?
An Immediately Invoked Function Expression is made by wrapping a function expression in parentheses and then invoking it immediately by adding another pair of parentheses after it. Here's an example:
(function() {
// This code will be executed immediately
})();
Without closure, any variable or function declared without a global reference will be deallocated after execution.
The advantage of using an IIFE is that it creates a new scope for your code, which helps to avoid conflicts with other scripts or global variables. Furthermore, because the code is self-contained, it can be used to create reusable modules or libraries.
Arguments can also be passed into an IIFE by adding them to the second set of parentheses. As an example:
(function(name) {
console.log('Hello ' + name);
})('World');
For example, to print the message "Hello World," we passed World as an argument.
Using IIFE in combined with DOM effects
This example takes me back to my first Javascript classes. The sample uses setInterval to change the background color at random, and the most important thing is that IIFE declares an Interval without leaving a trace, as scripts Analytics, ADS, and others do.
(function () {
setInterval(function () {
const number = Math.floor(Math.random() * 2)
document.body.style.backgroundColor = number === 1
? "yellow"
: "black";
}, 1000);
})();
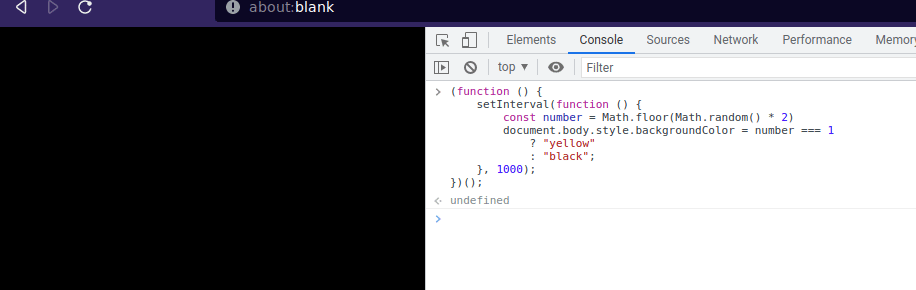
With Closures
We can initialize variables, share context with IIFE, and then use the value for an event, for example.
(function () {
let count = 0;
setInterval(function () {
console.log(`count=${count}`);
count++;
}, 1000)
})();
Named function
To track execution, IIFE can be named rather than anonymous.
(function myFunction(){
console.log(arguments.callee.name);
})();
Global Context
Yeap! IIFE allow us to define something in a global context, but keep in mind that having everything on global, as we did in the jQuery era, is not a good practice.
(function(){
class Foo {
say() { console.log("boo") }
}
globalThis.Foo = Foo;
// close friends' window.Foo = Foo
})();
const foo = new Foo();
foo.say()
// boo
That's all
So, hopefully, I've helped you understand or clarify something about JavasSript. Look at Google Analytics, Tag Manager Scripts, or JavaScript generated to see IIFE in action. God bless you and good day!
Time for feedback!