You must use Prettier as the code format for your TypeScript or JavaScript project.

I used to format my JavaScript by hand or with the Editor's default format, and sometimes I found myself disapproving the format code I had created a few minutes before; if this sounds familiar, it's time to change.
When I was a child, I spoke as a child, I understood as a child, I thought as a child: but when I became a man, I put away childish things. — 1 Corinthians 13:11 KJV.
Now that we are adults, it is time to add Prettier to our code; there are others in the game, yes! However, Prettier is essential for your project; simply trust.
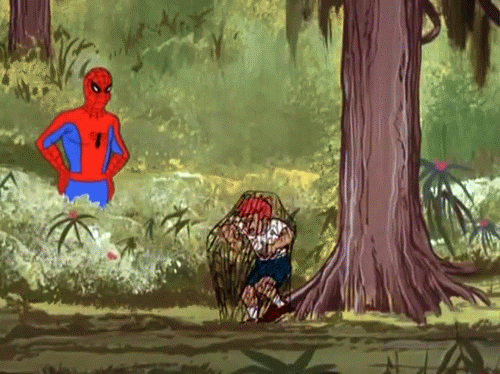
What is Prettier?
Prettier formats your code according to a set of rules. It supports a wide range of formatting options, such as indentation, line length, spacing, and others. Prettier also allows you to change the line length, use single or double quotes ❤️, and more.
Using Prettier on a daily basis can save developers a lot of time and effort by automatically formatting their code rather than having to manually make changes. As a result, at code-review, we will never fight over double quotes, single quotes, or commas.
The peace of God, which is deeper than all knowledge 🕊️
Prettier can be integrated into your favorite code editor, pipeline, or build process. It can be set to format automatically when we save a file or manually. Helping us in keeping up with the style throughout your code-base.
Installing Prettier
First, we must install prettier over NPM or Yarn; choose whatever works best for you!
npm install -D prettier
yarn add -D prettier
Setting up Prettier
Then we'll create a file called .prettierrc
to define our code style. Explore the official documentation to see available options.
{
"semi": false,
"singleQuote": true,
"printWidth": 80,
"tabWidth": 2,
"arrowParens": "avoid",
"trailingComma": "es5"
}
semi
- print semicolons at the ends of statements (true/false);singleQuote
- single quotes instead of double quotes (true/false);printWidth
- specify the line length that the printer will wrap on;tabWidth
- specify the number of spaces per indentation-level;arrowParens
- include or avoid parentheses around a sole arrow function parameter (avoid/always);trailingComma
- print trailing commas wherever possible in multi-line comma-separated syntactic structures (es5/none/all);
Take a look at Prettier settings in projects, for instance, React.
Testing
It's time to put the Prettier format to the test; I'll bring unformatted code that violate our code formatting guidelines.
export const printDoubleQuote = "eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9"
export const testPrintWidth = ( x ) => (x + 1) * (x +2) / (x + 3) + (x + 4 + 5 + 6)
export const testSemi = "ok";
export const testArrowParens = (x) => (y) => x * y * 2
export const testTrailingComma = [1, 2,]
With Prettier
export const printDoubleQuote = 'eyJhbGciOiJIUzI1NiIsInR5cCI6IkpXVCJ9'
export const testPrintWidth = x =>
((x + 1) * (x + 2)) / (x + 3) + (x + 4 + 5 + 6)
export const testSemi = 'ok'
export const testArrowParens = x => y => x * y * 2
export const testTrailingComma = [1, 2]
NPM script
We can write a npm
script to format all code, but I recommend using it on a pipeline or just to format all files related to pull requests.
- package.json
{
"scripts": {
"format": "prettier --write 'src/**/*.{js,jsx,ts,tsx,json}' --config ./.prettierrc"
}
}
The task will format any file in the (src) directory with the extensions (js, jsx, ts, tsx, json).
npm run format
VSCode integration
Before adding autoformat integration, you must first install the VSCode extension.
You should create a file name .vscode/settings.json
in your repository, or as global in your VSCode settings. json, but I wouldn't recommend making it global.
- .vscode/settings.json
{
"editor.formatOnSave": true
}
Finally, peacefulness during code review🕊️
More information is available at the Git repository.
I've brought you something useful to support you in establishing some basic rules for your code collaboration, specifically your code-style. In the following articles, we will set up eslint/tslint to check for code smells, type errors, and strange code ways.
Your kernel 🧠 has been updated! Bye, bye! 👍
Time for feedback!