How to use Bash default variables
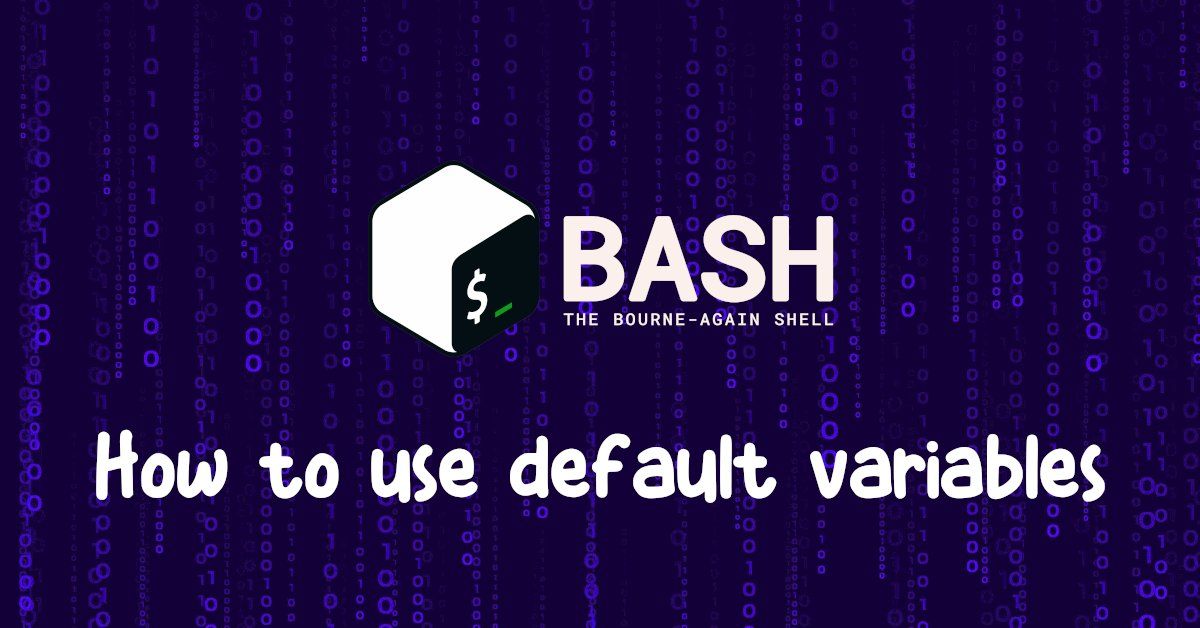
It's essential to have Bash scripts to support your servers or applications in a programming or DevOps environment. As a result, I'd like to share some Bash advice with you as variable default values.
Whatever operating system you are currently using. Linux is now used in everything from servers to containers to Raspberry Pi demo boards.
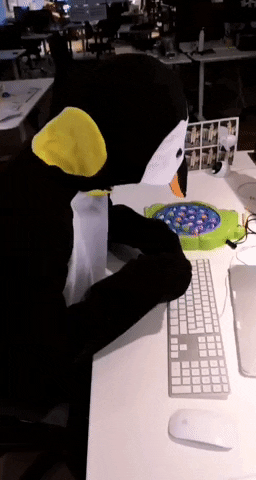
It's time to update your kernel, guys!
Sometimes you use variables to make your script more adaptable. For instance, I might use a variable named "OS_FAMILY" to indicate whether my script runs on Windows, Linux, RHEL, or Debian.
The OS_FAMILY variable will be used in the update system script.
First, it's time to write a regular script called update_system.bash.
Then, add and set the variable OS FAMILY.
#!/bin/bash
OS_FAMILY=$1
[[ ! -z "$OS_FAMILY" ]] && echo "Updating $OS_FAMILY..."
Next, let's check the variable and apply the expected behavior with case blocks.
#!/bin/bash
OS_FAMILY=$1
[[ ! -z "$OS_FAMILY" ]] && echo "Updating $OS_FAMILY..."
case $OS_FAMILY in
debian)
sudo apt update -y && sudo apt upgrade -y
;;
rhel)
sudo yum upgrade -y
;;
arch)
sudo pacman -Syu
;;
*)
echo "The script supports only (debian | rhel | arch)."
;;
esac
Okay, add Debian as the default value at line (3). "Sorry Manjaro 💔, I appreciate you, but I chose Debian flavors for my servers and containers."
#!/bin/bash
OS_FAMILY=${1-debian}
At this point, it's time to test the scripts.
Case 1. With argument;
bash bash_default_variables.bash "arch"
> Updating arch...
Case 2. With default value in action;
bash bash_default_variables.bash
> Updating debian...
Hmm, perhaps we can catch some bugs when the string is empty.
bash bash_default_variables.bash ""
> The script supports only (debian | rhel | arch).
Let's fix this at line (3).
#!/bin/bash
OS_FAMILY=${1:-debian}
All tests succeeded; the script is finished.
#!/bin/bash
OS_FAMILY=${1:-debian}
[[ ! -z "$OS_FAMILY" ]] && echo "Updating $OS_FAMILY..."
case $OS_FAMILY in
debian)
sudo apt update -y && sudo apt upgrade -y
;;
rhel)
sudo yum upgrade -y
;;
arch)
sudo pacman -Syu
;;
*)
echo "The script supports only (debian | rhel | arch)."
;;
esac
Guys, you now know how to use the default value in your bash scripts.
That's all folks.
Time for feedback!